A very good tool
http://vue-dev.uit.tufts.edu/index.cfm
Give it a try.
http://www.typealyzer.com/
The analysis indicates that the author of http://computingfunnyfacts.blogspot.com is of the type:
ISTP - The Mechanics
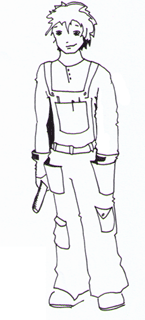
The Mechanics enjoy working together with other independent and highly skilled people and often like seek fun and action both in their work and personal life. They enjoy adventure and risk such as in driving race cars or working as policemen and firefighters.
Worlds you can create with Hex
First we have to generate all possible char combination:
From http://ribalba.de/geek/proj/hexword/wordcreate.py
1: #!/usr/bin/python
2:
3: #We want to create all possible combinations out of these chars
4: # a b c d e f ' '
5: #Somehow '' is not handeld correct so we have to remove it later
6: wl = ['a','b', 'c', 'd', 'e', 'f', ' ']
7: out = []
8:
9: def recLoop(txt):
10: if len(txt) >= len(wl):
11: #Add to list and remove space
12: out.append(txt.replace(' ',''))
13: else:
14: for i in set(wl):
15: recLoop(txt + i)
16:
17: #Start the recursion
18: recLoop("")
19:
20: #So we remove duplicates
21: outset = set(out)
22:
23: #And print
24: for w in set (outset):
25: print w
The output file can be found here : http://ribalba.de/geek/proj/hexword/outfile this file has 335923 lines and is 2.5M big. So now we have to find out how many words are actually 'real' English words. To make it easy I just used aspell and some perl.
Source can be found here: http://ribalba.de/geek/proj/hexword/findwords
1: #!/usr/bin/perlAnd this outputs us a nice list of all the words you can spell in English with the Hex chars, see here: http://ribalba.de/geek/proj/hexword/hexwords
2:
3: use Text::Aspell;
4:
5: my $speller = Text::Aspell->new;
6:
7: die unless $speller;
8:
9: # Set some options
10: $speller->set_option('sug-mode','fast');
11: $speller->set_option('clean-words','true');
12: $speller->set_option('ignore-case','true');
13:
14: open FILE, "outfile" or die $!;
15: while (my $line = <FILE>) {
16: chomp $line;
17: # check a word
18: if( $speller->check($line)) {
19: print $line . "\n";
20: }
21:
22: }
There are some freaky words there.
--------------------
CAFEBABE :)
Why recursion is so important
1: wl = ['a','b', 'c', 'd', 'e', 'f', '']But I think everyone can see that that was a stupid idea.
2: out = []
3:
4: for i1 in set(wl):
5: for i2 in set(wl):
6: for i3 in set(wl):
7: for i4 in set(wl):
8: for i5 in set(wl):
9: for i6 in set(wl):
10: for i7 in set(wl):
11: types = i1+i2+i3+i4+i5+i6+i7
12: out.append(types)
Saying that I have seen that in quite some projects. My next thought was to bring recursion into this.
1: wl = ['a','b', 'c', 'd', 'e', 'f', ' ']
2: out = []
3:
4: def recLoop(txt):
5: if len(txt) >= len(wl):
6: out.append(txt.replace(' ',''))
7: else:
8: for i in set(wl):
9: recLoop(txt + i)
This looks far nicer doesn't it. I hope everyone who programs can see this. Just to state how important recursion is and I don't really understand why unis don't teach this more.
lacheck port
Lacheck
LaCheck is a general purpose consistency checker for LaTeX documents.
It reads a LaTeX document and displays warning messages, if it finds
bad sequences. It should be noted, that the badness is very subjective.
LaCheck is designed to help find common mistakes in LaTeX documents,
especially those made by beginners.
Source RPM can be found at www.ribalba.de/geek/port/lacheck-1.26-1.src.rpm
Think multi core!
I normally write little bash scripts that do repeating jobs for me and I wrote a little script (simplified):
for i in `seq 100`; do ./ogp.py $i ; doneVery simple you would say. But where is the problem with this? This only uses one CPU core at a time. I normally work on a 2 core machine but I will be buying a 8 core soon. So this script only uses one. So now my script looks like this:
for i in `seq 100`; do ./ogp.py $i & doneCan you spot the difference. Now I background the job (&) and the loop continues to run. I will not clog up the process ques as the program only runs
$time ./ogp.py 1
real 0m0.034s
user 0m0.025s
sys 0m0.008s
But it makes some difference. No backgrounding:
$ time ./gentestAnd backgrounding
real 0m2.206s
user 0m1.479s
sys 0m0.727s
$ time ./gentest
real 0m1.128s
user 0m1.469s
sys 0m0.734s
So even with bash scripts you have to take the increase in core count serious.
Note: You don't need the line terminating ';' anymore as '&' acts as a line terminator too. So
for i in `seq 100`; do ./ogp.py $i &; doneWould be wrong
xpdf in CentOs
Download the source rpm here : xpdf-3.02-5.src.rpm
Thank you Carl for the nice spec file. Here is my extended one.
%define _prefix /usr
%define _version 3.02
Summary: open source viewer for Portable Document Format (PDF) files
Name: xpdf
Version: %{_version}
Release: 5
Source0: ftp://ftp.foolabs.com/pub/xpdf/xpdf-3.02.tar.gz
URL: http://www.foolabs.com/xpdf/
Group: Applications/Publishing
License: GPLv2
Patch0: ftp://ftp.foolabs.com/pub/xpdf/xpdf-3.02pl1.patch
Patch1: ftp://ftp.foolabs.com/pub/xpdf/xpdf-3.02pl2.patch
Patch2: patch-doc_sample-xpdfrc
Patch3: patch-xpdf_Object_h
BuildRoot: %{_topdir}/BUILD/%{name}-buildroot
Prefix: %{_prefix}
#Requires: t1lib
Requires: freetype > 2.0.5
Requires: htmlview
Requires: urw-fonts
Requires: xdg-utils
Requires: poppler-utils
Requires: xorg-x11-fonts-ISO8859-1-75dpi
BuildRequires: t1lib
BuildRequires: wxGTK
BuildRequires: t1lib-devel
BuildRequires: libpaper-devel
BuildPrereq: openmotif-devel
BuildPrereq: libX11-devel
BuildPrereq: freetype-devel >= 2.1.7
BuildPrereq: fileutils
%description
Xpdf is an open source viewer for Portable Document Format (PDF)
files. (These are also sometimes also called 'Acrobat' files, from
the name of Adobe's PDF software.) The Xpdf project also includes a
PDF text extractor, PDF-to-PostScript converter, and various other
utilities.
Xpdf runs under the X Window System on UNIX, VMS, and OS/2. The non-X
components (pdftops, pdftotext, etc.) also run on Win32 systems and
should run on pretty much any system with a decent C++ compiler.
Xpdf is designed to be small and efficient. It can use Type 1 or
TrueType fonts.
%prep
%setup -q
%patch -p1
%build
./configure --prefix=%{_prefix} --mandir=%{_mandir} --sysconfdir=/etc --with-freetype2-includes=/usr/include/freetype2
# ./configure \
# --prefix=%{_prefix}
# --mandir=%{_mandir}
# --sysconfdir=/etc
# --enable-multithreaded \
# --enable-wordlist \
# --with-x \
# --with-gzip \
# --enable-opi \
# # --with-appdef-dir=%{_datadir}/X11/app-defaults/ \
# --without-Xp-library \
# --with-t1-library \
# --with-freetype2-includes=/usr/include/freetype2/
make
%install
rm -rf $RPM_BUILD_ROOT
make DESTDIR=$RPM_BUILD_ROOT install
#poppler does this now. Stupid but works
rm $RPM_BUILD_ROOT%{_bindir}/pdffonts
rm $RPM_BUILD_ROOT%{_bindir}/pdfimages
rm $RPM_BUILD_ROOT%{_bindir}/pdfinfo
rm $RPM_BUILD_ROOT%{_bindir}/pdftops
rm $RPM_BUILD_ROOT%{_bindir}/pdftotext
rm $RPM_BUILD_ROOT%{_bindir}/pdftoppm
rm $RPM_BUILD_ROOT%{_mandir}/man1/pdffonts.1*
rm $RPM_BUILD_ROOT%{_mandir}/man1/pdfimages.1*
rm $RPM_BUILD_ROOT%{_mandir}/man1/pdfinfo.1*
rm $RPM_BUILD_ROOT%{_mandir}/man1/pdftops.1*
rm $RPM_BUILD_ROOT%{_mandir}/man1/pdftotext.1*
rm $RPM_BUILD_ROOT%{_mandir}/man1/pdftoppm.1*
%clean
rm -rf $RPM_BUILD_ROOT
%files
%defattr(-,root,root)
%doc ANNOUNCE COPYING CHANGES INSTALL README
%{_prefix}/bin/*
%{_mandir}/man1/*
%{_mandir}/man5/xpdfrc*
/etc/xpdfrc
%changelog
* Thu Nov 20 2008 Hoffmann Geerd-Dietger
- added 3.02pl2.patch
* Fri Aug 24 2007 Martin Brisby
- added 3.02pl1 patch
* Sat Jun 16 2007 Martin Brisby
- initial specfile
If you want to avoid the Pdf passwords I recommend to add this patch:
$OpenBSD: patch-xpdf_XRef_cc,v 1.4 2008/04/25 19:19:05 deanna Exp $
--- xpdf/XRef.cc.orig Thu Apr 24 19:13:00 2008
+++ xpdf/XRef.cc Thu Apr 24 19:50:06 2008
@@ -771,19 +771,19 @@ void XRef::setEncryption(int permFlagsA, GBool ownerPa
}
GBool XRef::okToPrint(GBool ignoreOwnerPW) {
- return (!ignoreOwnerPW && ownerPasswordOk) || (permFlags & permPrint);
+ return (1);
}
GBool XRef::okToChange(GBool ignoreOwnerPW) {
- return (!ignoreOwnerPW && ownerPasswordOk) || (permFlags & permChange);
+ return (1);
}
GBool XRef::okToCopy(GBool ignoreOwnerPW) {
- return (!ignoreOwnerPW && ownerPasswordOk) || (permFlags & permCopy);
+ return (1);
}
GBool XRef::okToAddNotes(GBool ignoreOwnerPW) {
- return (!ignoreOwnerPW && ownerPasswordOk) || (permFlags & permNotes);
+ return (1);
}
Object *XRef::fetch(int num, int gen, Object *obj) {
Hotmail vs. Gmail




What stops men from beeing Secretaries
- "They" think they can date easier. Its not really a secret that so called Nerds are not really good with girls and find it hard to date. I have talked to quite a lot of people that assured me the only reason they don't have a girlfriend is that there are not enough girls in IT. As this is a recurring argument I can only assume that this is one main reason.
- People are too politically correct, but through this, prove they are not. (Does this make sense?) Surly if you wouldn't be sexist you wouldn't care about the amount of girls. What difference does it make how many girls are in IT or not. I don't get it, through having an intended percentage of female employees, you are being even more than sexist, you are also being fascist.
To ensure equality and an increase in the female percentageI consider this to be sexist too. Now I don't wonder why people say things like "She only got the job because she is a girl" Writing stuff like this is only unfair to the girl that gets hired. Because everyone will assume she got hired to even out the percentage and she won't get a fair chance to prove what she really can. Though being overly correct they are harming the people they want to protect. I don't care who is doing the work, as long as he/she/it is doing good work, why would being of a sex, racial minority or anything determine if you get a job or not.
....
Females will be preferred, if equally qualified to other applicants.(translated)
I know this is highly controversial and I don't want to insult someone, but I just consider this practice to be wrong, deal with it.
Java md5 hash
For uni I wrote a little program that implements a sort of md5 hash. Just a 10 min program but quite nice and useful I would hope. It takes in a file and outputs the md5 sum and the file name. Used this to compare files on a computer with the version on a server. There must be better versions out there but it works
* See
* http://java.sun.com/j2se/1.4.2/docs/api/java/security/MessageDigest.html
* Basically a wrapper for the sun class
*/
import java.io.*;
import java.math.BigInteger;
import java.security.*;
import java.util.Scanner;
public class mdFive {
/**
* This function is passed a File name and it returns a md5 hash of
* this file.
* !!!! MADE STATIC SO I HAVE ONE FILE !!!!!
* !!!! NEVER DO THIS PLEASE REMOVE !!!!!
* @param FileToMd5
* @return The md5 string
*/
public static String md5File(String FileToMd5){
String outPutString = new String();
try {
MessageDigest algoToCrypt = MessageDigest.getInstance("MD5");
Scanner fileToCrypt = new Scanner(new File(FileToMd5));
/* Just to be on the safe side */
byte[] fileBuffer = null;
while( fileToCrypt.hasNextByte()){
algoToCrypt.update(fileBuffer, 0, fileToCrypt.nextByte());
}
/* Int would be to small and apparentelly you have to use a sign and magnitude */
BigInteger bigInt = new BigInteger(1, algoToCrypt.digest());
outPutString = bigInt.toString(16);
fileToCrypt.close();
} catch (Exception e) {
/* Not a big enough error to exit but still should tell someone */
System.err.println("Error while creating MD5 sum");
}
return outPutString;
}
/**
* The main programm
* @param args The arguments from the command line
*/
public static void main(String[] args) {
if(args.length == 0){
System.out.println("A MD5 Hascher \nUsage java mdFive
}else{
System.out.println( md5File(args[0]) + " , " + args[0]);
}
}
}
http://pages.google.com/unsupported
Our programming wizards tried their darndest to get Google Page Creator to work with as many browsers as possible. But alas, even the most expert practitioners of web sorcery must sleep now and again, lest their JavaScript magic run dry.
How people think my life looks like
To start with I kind of enjoyed being a participant. Especially with the horrible web page it was lots of fun because I could really shit about those stupid idiots who did the page. Probably they even call themselves IT/Web page experts. Those stupid people should be sent to the U.S.A.
Apart from that the web page is defiantly difficult to use for disabled people and women, especially the blonde ones with big breasts (even so I like them around me ).
But apart from a few things they are just useless as the web page is a well. Now one could think that those two would match perfectly but how I explained before they don't.
While I was testing the web page I drank a lot of tea, coffee and beer, which was probably the most enjoyable part as it usually is in life and not to forget the blond ladies out there.
Well what else can I say. The testing took me quite long because I had spent a lot of time on the toilet the weeks. First a will because the new FHM was really interesting and had lots of those “paticular women in it and I ate some bad bacon that one day.
What more to say :)
The first one walks in, takes a leak, and then proceeds to wash his hands. After washing them, he goes over to the paper dispenser, and takes reams and reams of paper. He then spends the next 10 minutes drying his hands, until there is no moisture left. As he walks out, he says to the others waiting, "At Microsoft, we're very thorough."
The next man walks in, takes a leak, then washes his hands. He then takes only 1 sheet of paper towel. He then dries his hands, making sure that he dries every single drop of water, using only one sheet of paper towel. After every single molecule of paper towel is soaked, and his hands are completely dry, he walks out, commenting that, "At Intel, we're thorough, and efficient."
Finally, the third engineer walks in, takes a leak, and then walks straight out again, saying, "At Sun, we don't piss on our hands."
Buzzword mania
Sudo
in the sudo inc_* file
/** HAL insults (paraphrased) from 2001.
*/
"Just what do you think you're doing Dave?",
"It can only be attributed to human error.",
"That's something I cannot allow to happen.",
"My mind is going. I can feel it.",
"Sorry about this, I know it's a bit silly.",
"Take a stress pill and think things over.",
"This mission is too important for me to allow you to jeopardize it.",
"I feel much better now.",
/*
* Insults from the original sudo(8).
*/
"Wrong! You cheating scum!",
"And you call yourself a Rocket Scientist!",
"No soap, honkie-lips.",
"Where did you learn to type?",
"Are you on drugs?",
"My pet ferret can type better than you!",
"You type like i drive.",
"Do you think like you type?",
"Your mind just hasn't been the same since the electro-shock, has it?",
/*
* CSOps insults (may be site dependent).
*/
"Maybe if you used more than just two fingers...",
"BOB says: You seem to have forgotten your passwd, enter another!",
"stty: unknown mode: doofus",
"I can't hear you -- I'm using the scrambler.",
"The more you drive -- the dumber you get.",
"Listen, broccoli brains, I don't have time to listen to this trash.",
"Listen, burrito brains, I don't have time to listen to this trash.",
"I've seen penguins that can type better than that.",
"Have you considered trying to match wits with a rutabaga?",
"You speak an infinite deal of nothing",
/*
* Insults from the "Goon Show."
*/
"You silly, twisted boy you.",
"He has fallen in the water!",
"We'll all be murdered in our beds!",
"You can't come in. Our tiger has got flu",
"I don't wish to know that.",
"What, what, what, what, what, what, what, what, what, what?",
"You can't get the wood, you know.",
"You'll starve!",
"... and it used to be so popular...",
"Pauses for audience applause, not a sausage",
"Hold it up to the light --- not a brain in sight!",
"Have a gorilla...",
"There must be cure for it!",
"There's a lot of it about, you know.",
"You do that again and see what happens...",
"Ying Tong Iddle I Po",
"Harm can come to a young lad like that!",
"And with that remarks folks, the case of the Crown vs yourself was proven.",
"Speak English you fool --- there are no subtitles in this scene.",
"You gotta go owwwww!",
"I have been called worse.",
"It's only your word against mine.",
"I think ... err ... I think ... I think I'll go home",
Howto validate A Email with regex
RFC 822 the standard
How to check if a email is valid as a regex
(?:(?:\r\n)?[ \t])*(?:(?:(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*))*@(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*))*|(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*)*\<(?:(?:\r\n)?[ \t])*(?:@(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*))*(?:,@(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*))*)*:(?:(?:\r\n)?[ \t])*)?(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*))*@(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*))*\>(?:(?:\r\n)?[ \t])*)|(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*)*:(?:(?:\r\n)?[ \t])*(?:(?:(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*))*@(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*))*|(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*)*\<(?:(?:\r\n)?[ \t])*(?:@(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*))*(?:,@(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*))*)*:(?:(?:\r\n)?[ \t])*)?(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*))*@(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*))*\>(?:(?:\r\n)?[ \t])*)(?:,\s*(?:(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*))*@(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*))*|(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*)*\<(?:(?:\r\n)?[ \t])*(?:@(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*))*(?:,@(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*))*)*:(?:(?:\r\n)?[ \t])*)?(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|"(?:[^\"\r\\]|\\.|(?:(?:\r\n)?[ \t]))*"(?:(?:\r\n)?[ \t])*))*@(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*)(?:\.(?:(?:\r\n)?[ \t])*(?:[^()<>@,;:\\".\[\] \x00-\x1F]+(?:(?:(?:\r\n)?[ \t])+|\Z|(?=[\["()<>@,;:\\".\[\]]))|\[([^\[\]\r\\]|\\.)*\](?:(?:\r\n)?[ \t])*))*\>(?:(?:\r\n)?[ \t])*))*)?;\s*)
Sweet isn't it
Geeks versus Nerds
After trying to explain to my mom what the differnece is here you go http://chickybaberules.blogspot.com/2006/04/chickybabes-guide-to-geeks-and-nerds.html
- Geeks are sociable people. Nerds have no social skills and are social outcasts.
- Geeks engage in meaningful conversations. They can look you in the eye, give you an unexpected timid smile and may even flirt with you. Nerds can’t maintain eye contact because they look at their gadgets and rattle on specifications. Note: The geek’s flirting methods may be ever so subtle, but it is still considered flirting.
- Geeks have a human touch, even during their geekiest moments, such as fixing your PC. Nerds don’t touch; they’re too busy flipping their pencils.
- Geeks can be sensitive, romantic and have a witty sense of humour. Nerds get romantic cutting code and playing with circuitboards.
- Geeks have style and a good fashion sense, even when they don’t have the physique for it. Nerds think that wearing a pen in your shirt pocket is the latest fashion accessory.
- Geeks can look cool in a suit if/when they wear one (ie job interviews). Nerds don’t look cool in anything.
- Geeks are sexy and can carry themselves with confidence. The words “nerds” and “sexy” are mutually exclusive.
- Geeks can be into sports and outdoor activities. Nerds are identified by pallor on their skin and loss of muscle tone; flipping pencils does not constitute a sport.
- Calling someone a geek can be a compliment; calling someone a nerd is derogatory.
- Geeks can be well-read and articulate. Nerds read hardware specs and installation guides for leisure.
- Geeks know they’re geeks, and they’re proud of it. Nerds don’t label themselves for fear of labels.
- Geeks and nerds have an inherent dislike of one another; I wonder why.
- Geeks can tell good jokes and laugh. Ever heard a nerd laugh?
- A nerd will offer to fix your computer for money. A geek will ask for other favours.
- Geeks take their girls to bed, nerds sleep at their desks.
- Geeks who talk in their sleep are likely to be dreaming of sex. Nerds who talk during their sleep speak of building computers*.
We love Sun
Gentoo / Emerge
if "moo" in myfiles:
print """
Gentoo (""" + os.uname()[0] + """)
_______________________
<>
-----------------------
\ ^__^
\ (oo)\_______
(__)\ )\/\
||----w |
|| ||
"""
spinner_msgs = ["Gentoo Rocks ("+os.uname()[0]+")",
"Thank you for using Gentoo. :)",
"Are you actually trying to read this?",
"How many times have you stared at this?",
"We are generating the cache right now",
"You are paying too much attention.",
"A theory is better than its explanation.",
"Phasers locked on target, Captain.",
"Thrashing is just virtual crashing.",
"To be is to program.",
"Real Users hate Real Programmers.",
"When all else fails, read the instructions.",
"Functionality breeds Contempt.",
"The future lies ahead.",
"3.1415926535897932384626433832795028841971694",
"Sometimes insanity is the only alternative.",
"Inaccuracy saves a world of explanation.",
]
I love Linux
who | grep -i blonde | date
cd ~; unzip; touch; strip; finger; head
mount; gasp; yes; uptime; umount
sleep
didi@alpha ~ $ \(-
bash: (-: command not found
Linux Kernel
/*
* Jeh, sometimes I really like the 386.
* This routine is called from an interrupt,
* and there should be absolutely no problem
* with sleeping even in an interrupt (I hope).
* Of course, if somebody proves me wrong, I'll
* hate intel for all time :-). We'll have to
* be careful and see to reinstating the interrupt
* chips before calling this, though.
*/
Linus
FitSpace security issue
... "We are glad you made it in last week." ...So how do they know this? When you go into the gym you have to swipe a RFID card. But surly they would not save this data with my name attaced to it. So let's say I would hack the system I would know when every member of the gym would visit it. Looking for somewhere on their web page (http://www.fitspacegyms.co.uk) to see what they are actually saving, I found that the page about the privacy police does not exist (http://www.fitspacegyms.co.uk/privacy-policy).
This feels a little like 1984. I can't wait till they send me advertisement suited to my training plan after FitSpace has made a lot of money selling this data to some other company. (This would not even be illegal)
"I, for one, welcome our new FitSpace overlords"
Another annoyance of Sun

I just wanted to download the new Solaris edition as I really like ZFS and I want it to be my native file system. But I have to logon to get to the download page. We are talking Sun here the company that is going to be bought or go bust soon. And the one thing they have everything riding on I can't even easily download. If I want to download ubuntu I can do this with two click from their start page ww.ubuntu.com
OK there might be some licensing issues here but please how do you expect me to use this if I am already pissed of before I have even downloaded the DVD. Don't get me wrong I love Sun and the stuff they do. But they just don't put in the extra mile. It seams to me they do all the fun stuff (ZFS, dtrace, etc ..) and then when they actually have to work towards the user they just give up.
According to a source, I have in the German government, they can't even make a offer for a super computer on time and in the specified budget. How will this end?

Why we love java
1 public static void main(String[] args) {What would you expect this to do? Like everyone with some sort of logical thinking mind you would assume that this would ask you for some sort of a String which you terminate with Enter then for a number with again you terminate with Enter and then another String. Run it and have a look. You will be amazed. It never asks you for the '3:' value? So why is this? Because if you call nextLine it will still leave some stuff in the buffer. This is then not returned by nextInt() as it is a String. So you are prompted to insert a int. But when it comes to the third read it will notice it still has something in it's buffer and return that. Even if there is nothing
2 Scanner sc = new Scanner(System.in);
3 System.out.println("1: ");
4 String l1 = sc.nextLine();
5 System.out.println("2: ");
6 int l2 = sc.nextInt();
7 System.out.println("3: ");
8 String l3 = sc.nextLine();
9 }
System.out.println("_" + l3 + "_");will open your eyes. You have to love the bad design of the Java libraries. We had something similar in ruby where we passed in a String that was not chomped to exists?
File.exists?("temp.txt\n")Another thing that caught us out in the scanner class is the hasNext. From the java source code of public boolean hasNext()
...So if there is something in the buffer return if not wait for input. That is really what you expect from this. I can see my self testing if there is something in the buffer with hasNext(). As this is what every sensible person would assume. But no, the people at Sun see this different. So what do we learn from this?
if (hasTokenInBuffer())
return revertState(true);
readInput();
...
=> Before anything look at the api documentation as there is no standard here and you will read
* Returns true if this scanner has another token in its input.
* This method may block while waiting for input to scan.
* The scanner does not advance past any input.
I love people stealing each other templates
Both have no copy right notice and I would have no idea who designed it. After some source code analysis I found out that this must be drupal. Have a look at http://drupal.org/node/212028
Does this look familiar. So Please if you use a theme at least quote who did it.
My talk at T-Dose
htop
it is amazing. Makes top sooo much nicer
Ubuntu is really helping Linux
OresteFri, Oct 17, 2008 at 10:06 AM
Reply-To: oregferra@yahoo.it
To: bug-coreutils@gnu.org, coreutils@gnu-org
I am new to Linux. 20 day ago I installed Ubuntu 8.04 and enjoyed it. 3 days ago I downloaded the recommended updates (120 Mega), after that I also installed Wine, a version found on a magazine I think it was 1.01 or similar).
Now when I start ubuntu, after the request of inserting name and password I receive the following message:
"user's $Home/.dmrc file being ignored. This prevents the default session and language from being saved. File should be owned by user and have 644 permissions. User's $Home directory must be owned by user and not writable by other users".
When I press OK, after a few seconds a second message appears:
"The Gnome session manager was unable to lock the file '/home/oreste/.Iceauthority! Please report this as a gnome bug"
And after that everything goes back to the request of name and password. I tried different sessions but with no result. Of course I do not know how to use the line commands.
Please Help
Oreste
I love quite a few things with this mail. But clearly the best are
- Of course I do not know how to use the line commands
- Please report this as a gnome bug
- Please Help
By the way here is a solution for your problem http://ubuntuforums.org/showthread.php?t=727677
or google is your friend.
Google generally does a great job, so they deserve their success wholeheartedly, but I have to tell you: Google's current position as the start page for the internet kind of scares the crap out of me, in a way that Microsoft's dominance over the desktop PC never did. I mean, monopoly power over a desktop PC is one thing -- but the internet is the whole of human knowledge, or something rapidly approaching that. Do we really trust one company to be a benevolent monopoly over.. well, everything?from http://www.codinghorror.com/blog/archives/001174.html
Markup Validation Service not used
Result: 654 Errors, 372 warning(s)Sweet. That can't all be my work and it is not. So next try, google.com
Result: 66 Errors, 8 warning(s)Ok, on the front page of Google there are 66 errors. No wonder my blog doesn't show up. And nearly all of them are absolutely stupid errors that could be fixed with just some little additions to the code. So if someone from Google is reading this you might be interested to fix this.
T-Dose website security issue
Add return codes to your shell prompt
export PS1='[\u@\h \w] $(echo $?) \$ 'the trick is the
$(echo $?)I know this forks a new echo process every time you get a shell prompt. But the idea is that you enable this when you are doing important selected stuff. Possibly on a live system where you are not allowed to make any errors.
Ahhh Google
Ruby on Rails with text_field_with_auto_complete
In my controller I need
# The first value is the model the second the name of the field
auto_complete_for :location, :name
# As of rails version > 2.0 forgery protection is activated by default. As the
# AJAX stuff won't work otherwise you have to disable it for one method
protect_from_forgery :except => [:auto_complete_for_location_name]
# This is the method called my the AJAX obj
def auto_complete_for_location_name
leg= params[:location].keys[0] # get index as its always only one at a time
auto_complete_responder_for_name params[:location][leg][:name]
end
# This does the real magic. It queries the database and then renders a
# partial 'names' with the list of values to display. With some sql knowledge
# you should be able to understand the query
private
def auto_complete_responder_for_name(value)
param= '%' + value.downcase + '%'
find_options= {
:conditions => [ 'LOWER(name) LIKE ?', param ],
:order => 'name ASC',
:limit => 10
}
@names = Location.find(:all, find_options)
render :partial => 'names'
end
So that is for the controller. Now we need to create the partial. You can call this what ever you want but for now I called it names. So you need a file named '_names'
<ul class="autocomplete_list">
<% for name in @names -%>
<li class="autocomplete_item"><%= name.name %> </li>
<% end -%>
</ul>
This is quite simple it get's the array @names we built in the controller and builds an unordered list out of the elements.
Next we have to add a little helper method to the app/helpers/application_helper.rb file. This is basically the wrapper for the 'real' auto_complete_field.
# Methods added to this helper will be available to all templates in the application.
module ApplicationHelper
def my_text_field_with_auto_complete(object, method, tag_options = {}, completion_options = {})
if(tag_options[:index])
tag_name = "#{object}_#{tag_options[:index]}_#{method}"
else
tag_name = "#{object}_#{method}"
end
(completion_options[:skip_style] ? "" : auto_complete_stylesheet) +
text_field(object, method, tag_options) +
content_tag("div", "", :id => tag_name + "_auto_complete", :class => "auto_complete") +
auto_complete_field(tag_name, { :url => { :action => "auto_complete_for_#{object}_#{method}" } }.update(completion_options))
end
end
So now we should have everything that is needed to create our little textbox. Now in the view you just add
<%= my_text_field_with_auto_complete :location, :name, {:index => '1'} %>And you increment the index value for every new field you add in that view.
Thank you to Wolfmans Howlings for posting all this.
http://blog.wolfman.com/articles/2006/10/17/having-multiple-text_field_with_auto_complete-in-the-same-view
http://wiki.rubyonrails.org/rails/pages/How+to+use+text_field_with_auto_complete
Hacking tip 2035
Why Debian is not Ubuntu and vice versa
(As of this writing, I'm still unaware of any bloat-free editions of Ubuntu.)beacuse that is debian.
Froscon 2008
http://programm.froscon.org/2008/events/208.de.html
The wiimote is a versatile and unique device as it is stuffed with electronic components that seldom are seen together. Because of this, the device has a myriad of uses and we will discuss one of them: using the wiimote as a universal presentation tool.
Hopefully, we can turn this presentation in a funny and amusing showcase of the possibilities of the Wiimote for giving presentations.
dnbradio
$ host 74.86.155.182
182.155.86.74.in-addr.arpa domain name pointer save.your.complaints.for.someone.who.gives-a-shit.com.
Cern Week x
My ldap cluster went out of warranty so we had to retire the machines. Installing the new cluster tunes out to be quite a pain. The idea was to have the new machines just take the templates from the old machines and reinstall them. But the new machines are blades and the install is not that easy. Because of not specifying the network interface in the kickstart file the install can not get the network interface. So all installs stop at boot and ask you which interface to use on the console. So I had to connect to every machine and tell it to use eth0. Now the next problem comes in that the machines will be using SLC5 and this is the first service to move to the new SLC version so loads of errors cropped up. Further when you reinstall a machine you totally forget how many little hacks you did while the machine was up that you didn't document. You just ssh in and change one value somewhere in a file. Now I was trying to remember what I did 7 month ago.
I also spent some time working on the Wii controller.
How true
From http://www.computerworld.com.au/index.php/id;1191304205;pp;3
Given GNU's desire to replicate Unix, I think Bison was inevitable. I am bemused that some GNU people are so irritated that GNU's contribution to Linux is not recognized, but yet they have failed to recognize their debt to those of us who worked on Unix...
Fun with stupid people

You know how sometimes some idiot tries to chat with you through msn and then tries to find out stuff about you. I was getting annoyed by these idiots as they kept trying to chat with me. So here a little chat I had today.
Conversation with gipuzkoa25cam@hotmail.com
(17:25:12) mmmmmmmmmmm: hola
(17:25:17) mmmmmmmmmmm: como te llamas?
(17:25:24) mmmmmmmmmmm has nudged you!
(17:28:48) didi: do I know you?
(17:29:18) mmmmmmmmmmm: you dont anderstand me?
(17:29:31) mmmmmmmmmmm: where are you from
(17:29:38) didi: who are you
(17:29:49) mmmmmmmmmmm: i´m david
(17:30:01) mmmmmmmmmmm: i´m from spain and you?
(17:31:32) Offering to send troy.exe to mmmmmmmmmmm
(17:31:48) mmmmmmmmmmm: no thanks
(17:31:53) mmmmmmmmmmm: is a troyan
(17:31:59) didi: you need this to communicate with me
(17:32:03) mmmmmmmmmmm: i´m not stupid
(17:32:04) didi: muhaaaaa
(17:32:34) mmmmmmmmmmm cancelled the transfer of troy.exe
(17:32:41) mmmmmmmmmmm has removed you from his or her buddy list.
The file was just
cat /dev/urandom > troy.exeby the way and you are stupid.
Democratic Linux
"We make it, you deal with it" + "By all means you can always fork it and make your own distro"
From : http://www.eubios.info/biodict.htm
DICTATORSHIP : When dictatorship relates to a mode of governing in modern states, it labels the unrestricted power of one person (or a group of individuals), who actually monopolizes and exercises all political powers. Dictators shape rules without being subjugated to them, and their actions cannot be sanctioned by anyone. All these features stand in sharp contrast to DEMOCRACY. Dictatorship can also refer to a particular mode of exercising power within a community or an ad hoc group of people, which is unrestrained by exterior forces and not dependent on the will formation within the group. (BP)
I think this fits pretty much all *NIX distros, I know of. So why has no one come up with the idea of having a democratic distro. Where the users can vote on what they want the developers to do. This of course might not be as fun for the developers, but surly you can get a better OS out of this.
So my idea is that with the install you get a little vote tool. Here you can get some information on what you can vote on and then you submit your vote to a central server. Through this the developers can get a good impression on what the community wants.
Of course there are some problems:
- How can you be sure no one has n virtual machines and votes n times
- What should you be able to vote for (People, package changes, etc ..)
- Should it be more like direct democracy or do you vote a representative every n months
- etc...
Cern Week 44
* sin2pwd
* perl in traint mde
* template migration, same tpl for SLC4 and SLC5
* new PrepareInstall tests
* Sourceforge migration
* ncm-accounts usermod fix (chomp missing)
That's it eBay
http://www.theregister.co.uk/2008/06/05/ebay_counterfeit_ruling/
Ok that is it for eBay then. The last real stand for it was that you could buy faked, stolen, cheep stuff of it. I think it is going to become site where a few people will sell a few old, collector style things
http://cgi.ebay.co.uk/ws/eBayISAPI.dll?ViewItem&item=260....5%26fvi%3D1
I might buy some parts for my motorbike from there because someone is selling out his garage. But I definitely will not buy something like a car, laptop, etc...
The stock market doesn't seam to think so. The last trade was 29.89 that is well in the 52wk Range: 25.10 - 40.73. I will follow up on thins. But I would predict that the shares will fall in the long run, if eBay doesn't through off a huge profit.
Https not supported
atime
$ ./atime.py 4 "sleep 0.5"
real 0.5
user 0.0
sys 0.0
for now you have to put the commands in quotes so it will pass in the whole command. Further be carefull not to loop to many times. Error catching works on a very rudenmental level.
$ ./atime.py 4 "xyz"
A error in the command has happend
/bin/sh: xyz: command not found
So if for your next assignment you need to take the average value of a program run time try atime.
1 #!/usr/bin/python
2 # A little tool that takes in a count and a command to
3 # run and averages the execution time
4 # ribalba@gmail.com
5
6 import sys;
7 import string;
8 import os;
9
10 av_real = av_user = av_sys= 0
11
12 looptimes = string.atoi(sys.argv[1])
13
14 for i in xrange(looptimes):
15 commandout = os.popen3("time -p " + sys.argv[2])
16 sys.stdout.write ( commandout[1].read(),);
17 steddout = commandout[2].readlines()
18 if (len (steddout) > 3):
19 sys.stderr.write("A error in the command has happend\n")
20 sys.stderr.write(steddout[0] + "\n")
21 sys.exit()
22 av_real += (string.atof(steddout[0].split()[1]) / looptimes)
23 av_user += (string.atof(steddout[1].split()[1]) / looptimes)
24 av_sys += (string.atof(steddout[2].split()[1]) / looptimes)
25 else:
26 sys.stderr.write("real " + str(av_real) + "\n")
27 sys.stderr.write("user " + str(av_user) + "\n")
28 sys.stderr.write("sys " + str(av_sys ) + "\n")
[DOWNLOAD]